Learning Vim as a Language: Nouns
The basics of the language of Vim commands.
One of the difficulties with learning how to use the Vim text editor is the number of commands that must be learned in order to edit text quickly. While only a minimal set of commands must be known to start using Vim, editing text will be more laborious than in a conventional editor until this set is expanded. Thus, a large scale memorization effort seems to be required before Vim becomes useful.
However, as is often the case, it is possible to sidestep a large amount of this memorization by learning some underlying theory. Once the basic theory of how Vim commands work is grasped, commands can be readily constructed as needed without much additional work. Only a small number of basic facts need to be known before much of the editor’s functionality becomes useable.
After learning how to switch editing modes, the next set of commands commonly
learned are for basic navigation: h
, j
, k
, l
. So we will start there.
Nouns
You can think of a Vim command as a sentence, containing a verb (the thing to do) and noun (the text to act upon). The verb is optional, and will default to “move cursor to” when left out. The movement commands then can be read as,
h move cursor to previous character
j move cursor to next line
k move cursor to previous line
l move cursor to next character
where the letter represents the “noun” and there is no specified verb. So, we can introduce four “nouns” into our Vim vocabulary immediately:
keystroke | description | type |
---|---|---|
h | previous character | noun |
j | next line | noun |
k | previous line | noun |
l | next character | noun |
You can experiment to see what text object within your document a given “noun” represents using Vim’s visual mode. This mode is typically considered to be a bad habit, however in this case it is quite nice for visualizing what different text objects look like.
You can enter visual mode by striking the v
key. When in visual mode, the
“move cursor to” action will be replaced by a “highlight” action. For example,
with the cursor beginning at the start of line 1023, striking the key sequence
v
, j
, j
, l
, l
, l
will result in the following highlighted text,
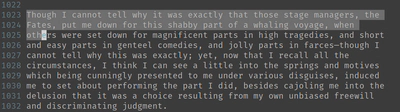
Notice that the end of this highlight is exactly where your cursor would have ended up had you issued these movement commands outside of visual mode. Leaving visual mode with ESC will clear the highlight and leave your cursor where it is.
Next word and previous word
Two other common “nouns” in the Vim language are w
and b
. These correspond
to “start of next word” and “start of previous word” respectively. These can
be easily remembered as w
for “word” and b
for “back [one word]”.
It is important to understand that these “nouns” refer to all text from where
the cursor currently is located, to the specified point. So, as an example, if
the cursor is located initially at the h in why on line 1027, w
will refer to
the highlighted text (not including the cursor location itself),
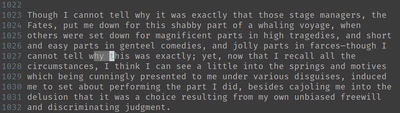
w
noun.
Likewise, with the cursor starting at the i in cunningly on line 1029, striking
the b
key in visual mode will result in the following text selection,
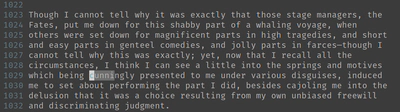
b
noun.
Notice here that, if the cursor is inside of a word, the b
key will consider
the “previous” word to be the current one. You can think of this as “moving
backwards until the start of a word is reached”.
You can also use the W
and B
keys for a similar purpose. These two “nouns”
refer to fundamentally the same thing, however they consider punctuation to be
part of a word, whereas w
and b
will stop upon encountering punctuation.
This distinction can be very useful when editing computer programs.
The “inside” modifier
The w
and b
nouns can be incredibly useful, especially for navigation
through a document. However, because they run from the cursor position until
the start/end of a word, they can be annoying to use when trying to select
entire words. You need to first navigate to the beginning of the word before
w
will correctly refer to the entire word itself, as is the case in this
example of selecting the word “performing” on line 1031. The cursor must be
on the p at the start of the word (not even the space before it will work) to
get the following highlight,
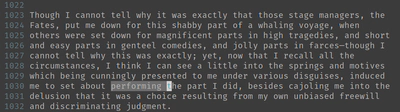
vw
Note that this selection includes both the space after the word, and the
first letter of the next word. If you want only the word then the cursor
would need to be on the g at the end of the word, and then the word selected
with b
, as is the case here,
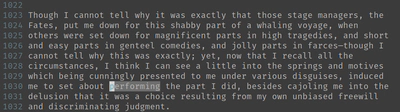
vb
As you can see, selecting an entire word, and only that word, is a little
counter-intuitive, as it requires navigating to its end, and using b
. This
doesn’t matter much for movement, and we will see in the future article on
verbs that many of these also do not have these issues when combined with
w
and b
, however if your goal is to select a specific word in visual mode,
then this solution is a bit clunky.
For this reason, Vim has nouns that allow you to refer to an entire text object
that contains the cursor. These nouns are actually a two character sequence,
beginning with i
.
For example, to select an entire word, move the cursor to anywhere inside of
the word, and then (from visual mode) strike iw
, which can be read as “inside
word”. With the cursor anywhere inside of the word performing on line 1030, you
will get the following selection,
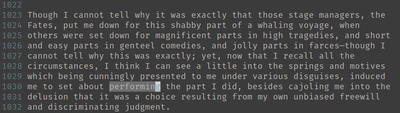
viw
No such combination exists for b
.
Notice that these “nouns” cannot be used for movement. As a movement command
contains no preceding “verb”, using iw
alone in normal/command mode would
result in entering insert mode and typing a w. This makes practical sense, as
these “inner nouns” don’t make much sense in the context of movement. “Move the
cursor to the word containing the cursor” is a task already accomplished with
w
and b
.
Other Nouns
Aside from words, there are a few other common nouns that can be used. Most of
these are of the “inner” variety, and cannot be used in the absence of the i
.
$ -- to the end of the current line
^ -- to the beginning of the current line
ip -- the entire paragraph containing the cursor
is -- the entire sentence containing the cursor
i" -- if the cursor is inside of quotation marks, all of the text between them
i( -- if the cursor is inside of parenthese, all of the text between them
i[ -- if the cursor is inside of brackets, all of the text between them
i{ -- if the cursor is inside of braces, all of the text between them
i' -- if the cursor is inside of single quotes, all of the text between them
i` -- if the cursor is inside of backticks, all of the text between them
As you can imagine, the last several of these are very useful for programming, where the various symbols mention are used quite frequently. If the cursor is not currently inside parentheses, backticks, etc., then the corresponding noun in the list will simply refer to nothing.
As an example of these new nouns, consider this function definition:
int foo(int bar, char *baz)
{
...
}
The noun i(
can be used to refer to the entire parameter list, and the
noun i{
can be used to refer to the entire body of the function. These can
be read as “all the text inside of the parentheses” and “all the text inside of
the braces” respectively.
Conclusion
In this post, we talked about a number of “nouns” within the Vim language. That is to say, we learned Vim commands that correspond to selecting sections of text to operate upon. By default, these are often interpreted as movement commands, or are used for selecting text in visual mode, as we did here. In the next article in this series, we’ll talk about “verbs”–various Vim commands that will allow us to operate upon these defined chunks of text.